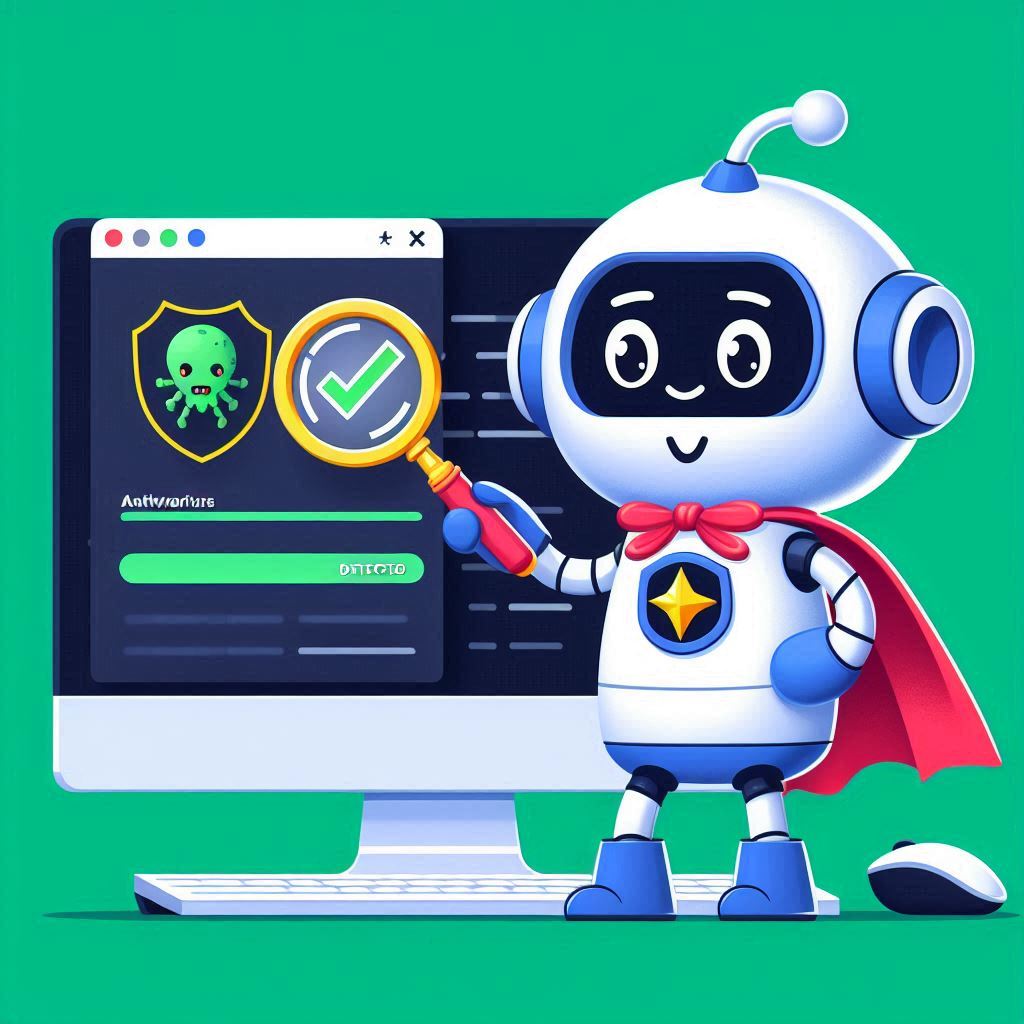
Norton Antivirus, developed by NortonLifeLock, is one of the most trusted and widely used security tools for detecting and neutralizing malicious software. Its virus detection methods are built on a combination of traditional and advanced techniques, offering robust protection against evolving threats. In this article, we will dive into the methods Norton employs for detecting viruses, with a focus on the technical algorithms and approaches behind these techniques. We will also include C language examples and pseudocode to provide a deeper understanding for developers.
Signature-Based Detection
The most fundamental method of virus detection used by Norton is signature-based detection. This method involves comparing the code of scanned files with a database of known malware signatures. A signature is a specific pattern of bytes or code sequences that uniquely identifies a virus.
Example of Signature Matching Algorithm in C
include
include
int matchSignature(const char *fileData, const char *virusSignature) {
if (strstr(fileData, virusSignature) != NULL) {
return 1; // Virus signature found
}
return 0; // No match
}
int main() {
const char *fileData = « This is a sample file with malicious code… »;
const char *virusSignature = « malicious code »;
if (matchSignature(fileData, virusSignature)) {
printf("Virus detected!\n");
} else {
printf("File is clean.\n");
}
return 0;
}
In this simple example, we check if a file contains a particular signature. Of course, in real-world scenarios, virus signatures are far more complex, and Norton uses an extensive and constantly updated database to ensure that all known threats are accounted for.
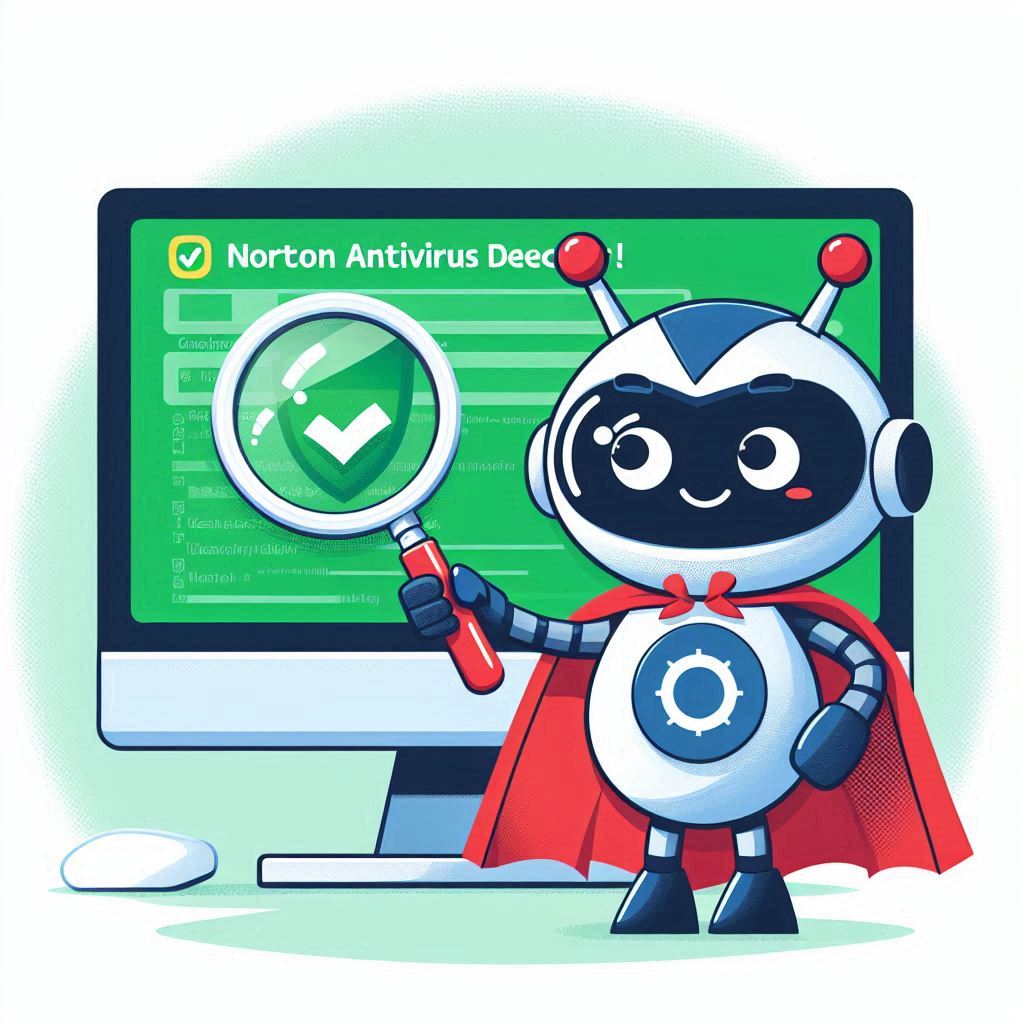
Heuristic Analysis
Heuristic analysis is used by Norton to detect previously unknown or new variations of viruses. This method looks for suspicious patterns or behaviors in the code, even if the malware does not match any known signatures. The goal is to identify files that exhibit characteristics typical of viruses, such as abnormal file modifications or suspicious system calls.
Pseudocode for Heuristic Analysis
// Pseudocode for heuristic-based virus detection
function heuristicAnalysis(file):
score = 0
if file.modifySystemFiles():
score += 2
if file.requestsElevatedPrivileges():
score += 2
if file.executesObfuscatedCode():
score += 1
if score > threshold:
return « Potential threat »
else:
return « File is clean »
In the heuristic approach, the file is assigned a « suspicion score » based on its behavior. If the score exceeds a certain threshold, Norton flags the file as potentially malicious. This method is crucial for detecting zero-day threats, where viruses mutate to evade signature-based detection.
Behavioral Detection
Behavioral detection is another advanced method used by Norton to identify malware by monitoring a program’s actions in real time. Rather than analyzing the static code of a file, this method tracks how the program behaves after execution. Suspicious activities, such as attempting to modify system files, accessing sensitive user data, or making unauthorized network requests, are flagged and analyzed for possible malicious intent.
Behavioral detection is particularly effective against polymorphic viruses, which modify their appearance or code signature to evade traditional detection methods. By focusing on what the program does rather than how it looks, Norton can detect and block even well-camouflaged threats.
Pseudocode for Behavioral Detection
// Pseudocode for runtime behavioral detection
function monitorProgramExecution(program):
while program.isRunning():
if program.accessesSystemFiles():
logWarning(« Suspicious access to system files. »)
if program.altersRegistryKeys():
logWarning(« Potential malware: modifying registry. »)
if program.sendsDataToUnknownIP():
logWarning(« Suspicious network activity detected. »)
if warningCount > threshold:
return « Malware behavior detected »
return « No threat detected »
This simplified pseudocode simulates monitoring a running program’s behavior. If suspicious actions accumulate to a certain threshold, the program is flagged as a threat.
Cloud-Based Detection
Norton enhances its virus detection capabilities with cloud-based analysis. When a potential threat is detected, the information is uploaded to Norton’s cloud for real-time analysis. Here, the file is compared against the latest malware signatures, behavioral patterns, and heuristic rules stored in Norton’s vast cloud database.
This approach leverages crowdsourced intelligence from millions of devices, ensuring that emerging threats are quickly detected and neutralized. The cloud-based system enables faster and more accurate detection by utilizing the collective knowledge of Norton’s global user base.
Cloud-Based Detection Workflow
- File is scanned locally – if a potential threat is found, the file is flagged for further analysis.
- Upload to the cloud – the suspicious file or behavior is sent to Norton’s cloud servers for in-depth analysis.
- Cloud-based analysis – the file is analyzed using the latest signatures, heuristics, and behavioral rules.
- Response – if the file is confirmed as malware, the user is notified, and the threat is quarantined or removed.
- Machine Learning Algorithms
Norton employs machine learning algorithms to improve virus detection accuracy. These algorithms can analyze vast amounts of data, learn from patterns, and identify emerging threats based on subtle variations in code or behavior. Machine learning is particularly useful for detecting advanced persistent threats (APTs) that evolve over time or adapt to evade detection.
Machine Learning Model Example
// Pseudocode for a simplified machine learning model
function trainModel(trainingData):
model = initializeModel()
for each data in trainingData:
model.learn(data.features, data.label)
return model
function classifyNewFile(file, model):
features = extractFeatures(file)
prediction = model.predict(features)
if prediction == « malware »:
return « Virus detected »
else:
return « File is clean »
Machine learning-based detection helps Norton stay ahead of threats by continually learning from both existing and newly encountered malware samples.
Conclusion
Norton Antivirus uses a combination of traditional and modern virus detection techniques, including signature-based detection, heuristic analysis, behavioral detection, cloud-based analysis, and machine learning algorithms. Together, these methods provide comprehensive protection against known and emerging threats, ensuring that users are safeguarded from viruses, malware, and advanced cyberattacks.
By integrating real-time monitoring and advanced analytics, Norton not only detects but also preemptively blocks malicious software before it can harm users’ systems.